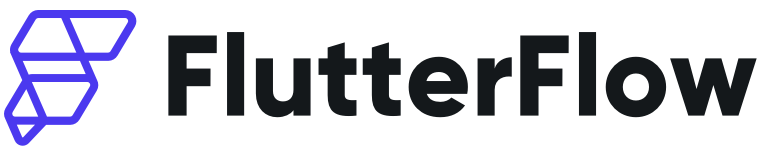
Visual Development Platform
FlutterFlow is a visual development platform that lets you build mobile, web, and desktop apps incredibly fast, without sacrificing quality or features.
Before You Begin
Ensure you meet system requirements and grasp technical concepts for smooth building in FlutterFlow.
Explore FlutterFlow
Dive into the building blocks of the platform: projects, widgets, functions and more.
Start Building
Jump right into a quick start on how to build a simple app.
What's New in FlutterFlow
Updates, features, and the latest enhancements in FlutterFlow.
Community Forum
Join discussions, ask questions, and share insights with the FlutterFlow community.
Submit Bug Report
Encountered a bug in FlutterFlow? Help us improve by submitting a bug report.